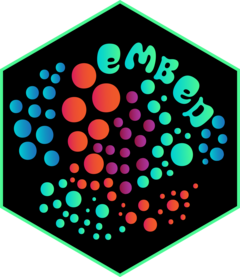
Entity Embeddings of Categorical Variables using TensorFlow
Source:vignettes/Applications/Tensorflow.Rmd
Tensorflow.Rmd
The approach encodes categorical data as multiple numeric variables using a word embedding approach. Originally intended as a way to take a large number of word identifiers and represent them in a smaller dimension. Good references on this are Guo and Berkhahn (2016) and Chapter 6 of Francois and Allaire (2018).
The methodology first translates the C factor levels as a
set of integer values then randomly allocates them to the new D
numeric columns. These columns are optionally connected in a neural
network to an intermediate layer of hidden units. Optionally, other
predictors can be added to the network in the usual way (via the
predictors
argument) that also link to the hidden layer.
This implementation uses a single layer with ReLu activations. Finally,
an output layer is used with either linear activation (for numeric
outcomes) or softmax (for classification).
To translate this model to a set of embeddings, the coefficients of the original embedding layer are used to represent the original factor levels.
As an example, we use the Ames housing data where the sale price of houses are being predicted. One predictor, neighborhood, has the most factor levels of the predictors.
library(tidymodels)
data(ames)
length(levels(ames$Neighborhood))
## [1] 29
The distribution of data in the neighborhood is not uniform:
ames %>%
count(Neighborhood) %>%
ggplot(aes(n, reorder(Neighborhood, n))) +
geom_col() +
labs(y = NULL) +
theme_bw()
Fo plotting later, we calculate the simple means per neighborhood:
means <-
ames %>%
group_by(Neighborhood) %>%
summarise(
mean = mean(log10(Sale_Price)),
n = length(Sale_Price),
lon = median(Longitude),
lat = median(Latitude)
)
We’ll fit a model with 10 hidden units and 3 encoding columns:
library(embed)
tf_embed <-
recipe(Sale_Price ~ ., data = ames) %>%
step_log(Sale_Price, base = 10) %>%
# Add some other predictors that can be used by the network
# We preprocess them first
step_YeoJohnson(Lot_Area, Full_Bath, Gr_Liv_Area) %>%
step_range(Lot_Area, Full_Bath, Gr_Liv_Area) %>%
step_embed(
Neighborhood,
outcome = vars(Sale_Price),
predictors = vars(Lot_Area, Full_Bath, Gr_Liv_Area),
num_terms = 5,
hidden_units = 10,
options = embed_control(epochs = 75, validation_split = 0.2)
) %>%
prep(training = ames)
theme_set(theme_bw() + theme(legend.position = "top"))
tf_embed$steps[[4]]$history %>%
filter(epochs > 1) %>%
ggplot(aes(x = epochs, y = loss, col = type)) +
geom_line() +
scale_y_log10()
The embeddings are obtained using the tidy
method:
hood_coef <-
tidy(tf_embed, number = 4) %>%
dplyr::select(-terms, -id) %>%
dplyr::rename(Neighborhood = level) %>%
# Make names smaller
rename_at(
vars(contains("emb")),
funs(gsub("Neighborhood_", "", ., fixed = TRUE))
)
hood_coef
## # A tibble: 30 × 6
## embed_1 embed_2 embed_3 embed_4 embed_5 Neighborhood
## <dbl> <dbl> <dbl> <dbl> <dbl> <chr>
## 1 -0.0455 -0.0389 -0.0212 0.00785 0.0172 ..new
## 2 -0.0553 -0.00705 0.0422 -0.0445 0.0406 North_Ames
## 3 -0.0440 -0.0465 -0.00791 -0.0261 -0.0387 College_Creek
## 4 0.0498 0.0146 -0.00975 -0.0201 0.0785 Old_Town
## 5 0.0362 -0.0135 0.0471 0.0297 0.0471 Edwards
## 6 -0.00750 -0.0576 0.0103 -0.0164 -0.112 Somerset
## 7 -0.0541 -0.146 -0.00780 -0.0661 -0.116 Northridge_Heights
## 8 -0.0399 -0.00227 0.0274 0.0308 -0.0191 Gilbert
## 9 0.00240 0.0114 0.0509 -0.0731 0.0170 Sawyer
## 10 0.0288 -0.0579 -0.0349 0.0161 0.00614 Northwest_Ames
## # ℹ 20 more rows
hood_coef <-
hood_coef %>%
inner_join(means, by = "Neighborhood")
hood_coef
## # A tibble: 28 × 10
## embed_1 embed_2 embed_3 embed_4 embed_5 Neighborhood mean n
## <dbl> <dbl> <dbl> <dbl> <dbl> <chr> <dbl> <int>
## 1 -0.0553 -0.00705 0.0422 -0.0445 0.0406 North_Ames 5.15 443
## 2 -0.0440 -0.0465 -0.00791 -0.0261 -0.0387 College_Creek 5.29 267
## 3 0.0498 0.0146 -0.00975 -0.0201 0.0785 Old_Town 5.07 239
## 4 0.0362 -0.0135 0.0471 0.0297 0.0471 Edwards 5.09 194
## 5 -0.00750 -0.0576 0.0103 -0.0164 -0.112 Somerset 5.35 182
## 6 -0.0541 -0.146 -0.00780 -0.0661 -0.116 Northridge_Hei… 5.49 166
## 7 -0.0399 -0.00227 0.0274 0.0308 -0.0191 Gilbert 5.27 165
## 8 0.00240 0.0114 0.0509 -0.0731 0.0170 Sawyer 5.13 151
## 9 0.0288 -0.0579 -0.0349 0.0161 0.00614 Northwest_Ames 5.27 131
## 10 -0.00468 -0.0322 0.0528 -0.0397 -0.00754 Sawyer_West 5.25 125
## # ℹ 18 more rows
## # ℹ 2 more variables: lon <dbl>, lat <dbl>
We can make a simple, interactive plot of the new features versus the outcome:
tf_plot <-
hood_coef %>%
dplyr::select(-lon, -lat) %>%
gather(variable, value, starts_with("embed")) %>%
# Clean up the embedding names
# Add a new variable as a hover-over/tool tip
mutate(
label = paste0(gsub("_", " ", Neighborhood), " (n=", n, ")"),
variable = gsub("_", " ", variable)
) %>%
ggplot(aes(x = value, y = mean)) +
geom_point_interactive(aes(size = sqrt(n), tooltip = label), alpha = .5) +
facet_wrap(~variable, scales = "free_x") +
theme_bw() +
theme(legend.position = "top") +
labs(y = "Mean (log scale)", x = "Embedding")
girafe(ggobj = tf_plot)
However, this has induced some between-predictor correlations:
## embed_1 embed_2 embed_3 embed_4 embed_5
## embed_1 1.00 0.24 0.15 0.16 0.48
## embed_2 0.24 1.00 0.16 0.17 0.52
## embed_3 0.15 0.16 1.00 0.10 0.29
## embed_4 0.16 0.17 0.10 1.00 0.19
## embed_5 0.48 0.52 0.29 0.19 1.00